Going Through Javascript Basics Again After Finishing It Freecodecamp
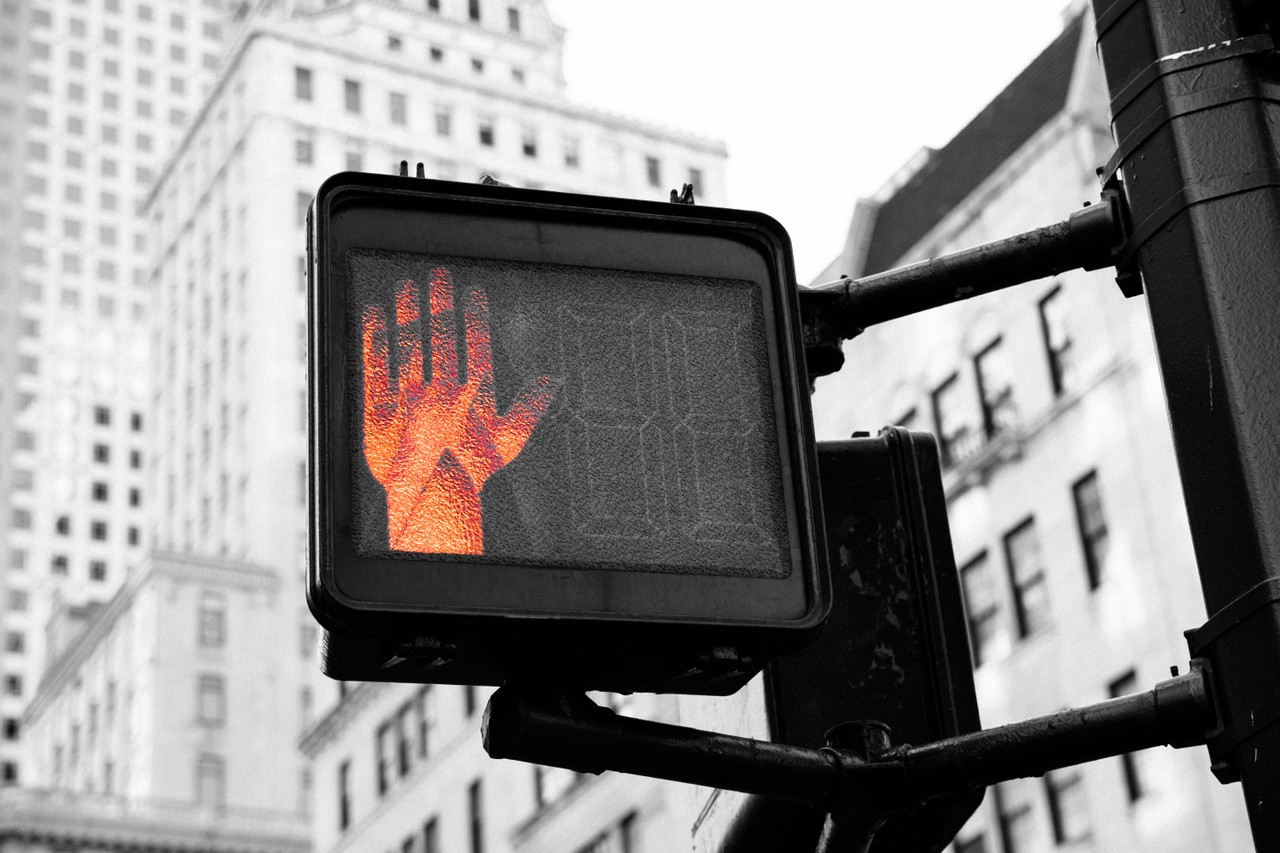
When does an asynchronous function finish? And why is this such a hard question to answer?
Well it turns out that understanding asynchronous functions requires a great deal of cognition about how JavaScript works fundamentally.
Let's go explore this concept, and learn a lot most JavaScript in the process.
Are yous ready? Allow's go.
What is asynchronous lawmaking?
By blueprint, JavaScript is a synchronous programming language. This means that when code is executed, JavaScript starts at the height of the file and runs through code line by line, until information technology is done.
The issue of this design conclusion is that only i thing can happen at whatever one time.
You can think of this as if you were juggling six small-scale assurance. While y'all are juggling, your easily are occupied and can't handle anything else.
It's the aforementioned with JavaScript: once the code is running, it has its hands full with that code. We call this this kind of synchronous code blocking. Because information technology'due south finer blocking other code from running.
Let's circle back to the juggling example. What would happen if you lot wanted to add another brawl? Instead of six assurance, you wanted to juggle seven balls. That's might be a problem.
You don't want to stop juggling, considering information technology'due south only so much fun. Just y'all can't go and become another ball either, because that would mean you'd take to stop.
The solution? Consul the work to a friend or family member. They aren't juggling, so they can get and become the brawl for you, then toss it into your juggling at a time when your hand is free and you are ready to add another ball mid-juggle.
This is what asynchronous code is. JavaScript is delegating the work to something else, and so going about information technology's own business. Then when it'southward ready, information technology volition receive the results back from the piece of work.
Who is doing the other work?
Alright, then we know that JavaScript is synchronous and lazy. It doesn't want to exercise all of the work itself, and so it farms it out to something else.
Merely who is this mysterious entity that works for JavaScript? And how does it get hired to work for JavaScript?
Well, permit's take a wait at an example of asynchronous code.
const logName = () => { panel.log("Han") } setTimeout(logName, 0) console.log("Hi there")
Running this code results in the following output in the panel:
// in console Hi there Han
Alright. What is going on?
Information technology turns out that the way we farm out work in JavaScript is to use environment-specific functions and APIs. And this is a source of great confusion in JavaScript.
JavaScript always runs in an surround.
Often, that surround is the browser. Only it tin can as well be on the server with NodeJS. Just what on earth is the difference?
The divergence – and this is important – is that the browser and the server (NodeJS), functionality-wise, are not equivalent. They are often like, but they are non the aforementioned.
Let'southward illustrate this with an case. Let's say JavaScript is the protagonist of an epic fantasy volume. Merely an ordinary farm child.
At present allow's say that this farm kid found two suits of special armor that gave them powers beyond their own.
When they used the browser suit of armor, they gained access to a certain set of capabilities.
When they used the server suit of armor they gained access to another set up of capabilities.
These suits have some overlap, because the creators of these suits had the same needs in certain places, but not in others.
This is what an environs is. A place where lawmaking is run, where there exist tools that are built on top of the existing JavaScript language. They are not a part of the language, but the line is oft blurred considering we use these tools every day when nosotros write code.
setTimeout, fetch, and DOM are all examples of Web APIs. (You tin see the full list of Web APIs here.) They are tools that are built into the browser, and that are made available to u.s.a. when our lawmaking is run.
And because we ever run JavaScript in an surroundings, it seems like these are part of the linguistic communication. But they are not.
So if y'all've ever wondered why yous can use fetch in JavaScript when you run information technology in the browser (just need to install a package when you run it in NodeJS), this is why. Someone thought fetch was a good thought, and built it every bit a tool for the NodeJS environs.
Confusing? Yes!
But now we can finally understand what takes on the work from JavaScript, and how it gets hired.
It turns out that information technology is the environment that takes on the work, and the fashion to get the environment to do that work, is to use functionality that belongs to the environment. For example fetch or setTimeout in the browser surroundings.
What happens to the work?
Slap-up. So the surroundings takes on the work. Then what?
At some point you lot need to get the results back. Simply let'southward call up about how this would piece of work.
Let's go back to the juggling example from the beginning. Imagine you lot asked for a new ball, and a friend just started throwing the ball at you lot when you weren't prepare.
That would be a disaster. Maybe you could get lucky and catch information technology and get it into your routine effectively. Simply theres a big chance that information technology may crusade you to drop all of your balls and crash your routine. Wouldn't it be better if you gave strict instructions on when to receive the ball?
As it turns out, there are strict rules surrounding when JavaScript can receive delegated piece of work.
Those rules are governed by the outcome loop and involve the microtask and macrotask queue. Yes, I know. It's a lot. But bear with me.

Alright. So when we consul asynchronous code to the browser, the browser takes and runs the code and takes on that workload. But in that location may be multiple tasks that are given to the browser, and then nosotros need to make sure that we tin prioritise these tasks.
This is where the microtask queue and the macrotask queue come in play. The browser will take the work, do it, then place the result in one of the two queues based on the type of work information technology receives.
Promises, for example, are placed in the microtask queue and have a higher priority.
Events and setTimeout are examples of work that is put in the macrotask queue, and have a lower priority.
Now one time the work is done, and is placed in one of the ii queues, the result loop will run back and forth and cheque whether or not JavaScript is ready to receive the results.
But when JavaScript is done running all its synchronous code, and is practiced and ready, volition the event loop showtime picking from the queues and handing the functions dorsum to JavaScript to run.
So let's take a look at an case:
setTimeout(() => console.log("howdy"), 0) fetch("https://someapi/information").and then(response => response.json()) .and then(data => panel.log(information)) console.log("What soup?")
What will the lodge be here?
- Firstly, setTimeout is delegated to the browser, which does the work and puts the resulting function in the macrotask queue.
- Secondly fetch is delegated to the browser, which takes the piece of work. It retrieves the information from the endpoint and puts the resulting functions in the microtask queue.
- Javascript logs out "What soup"?
- The event loop checks whether or non JavaScript is ready to receive the results from the queued work.
- When the console.log is done, JavaScript is set. The event loop picks queued functions from the microtask queue, which has a higher priority, and gives them back to JavaScript to execute.
- After the microtask queue is empty, the setTimeout callback is taken out of the macrotask queue and given back to JavaScript to execute.
In console: // What soup? // the data from the api // hi
Promises
Now y'all should have a practiced deal of knowledge most how asynchronous lawmaking is handled by JavaScript and the browser surroundings. So let's talk about promises.
A promise is a JavaScript construct that represents a hereafter unknown value. Conceptually, a hope is just JavaScript promising to return a value. Information technology could exist the result from an API phone call, or it could be an error object from a failed network request. Y'all're guaranteed to become something.
const hope = new Hope((resolve, turn down) => { // Make a network request if (response.status === 200) { resolve(response.body) } else { const mistake = { ... } reject(error) } }) hope.and so(res => { console.log(res) }).catch(err => { panel.log(err) })
A promise can have the following states:
- fulfilled - action successfully completed
- rejected - action failed
- pending - neither action has been completed
- settled - has been fulfilled or rejected
A promise receives a resolve and a reject function that tin exist called to trigger one of these states.
One of the big selling points of promises is that we can chain functions that we desire to happen on success (resolve) or failure (reject):
- To register a function to run on success we use .and then
- To register a role to run on failure nosotros utilize .catch
// Fetch returns a hope fetch("https://swapi.dev/api/people/ane") .and then((res) => console.log("This office is run when the request succeeds", res) .grab(err => console.log("This office is run when the request fails", err) // Chaining multiple functions fetch("https://swapi.dev/api/people/ane") .then((res) => doSomethingWithResult(res)) .and then((finalResult) => console.log(finalResult)) .catch((err => doSomethingWithErr(err))
Perfect. At present let's have a closer wait at what this looks like under the hood, using fetch every bit an example:
const fetch = (url, options) => { // simplified return new Promise((resolve, decline) => { const xhr = new XMLHttpRequest() // ... make asking xhr.onload = () => { const options = { status: xhr.status, statusText: xhr.statusText ... } resolve(new Response(xhr.response, options)) } xhr.onerror = () => { reject(new TypeError("Request failed")) } } fetch("https://swapi.dev/api/people/one") // Register handleResponse to run when promise resolves .then(handleResponse) .catch(handleError) // conceptually, the promise looks similar this now: // { status: "pending", onsuccess: [handleResponse], onfailure: [handleError] } const handleResponse = (response) => { // handleResponse will automatically receive the response, ¨ // because the hope resolves with a value and automatically injects into the part console.log(response) } const handleError = (response) => { // handleError will automatically receive the mistake, ¨ // because the promise resolves with a value and automatically injects into the function console.log(response) } // the promise will either resolve or refuse causing it to run all of the registered functions in the respective arrays // injecting the value. Let'south inspect the happy path: // 1. XHR outcome listener fires // 2. If the request was successfull, the onload outcome listener triggers // three. The onload fires the resolve(VALUE) part with given value // four. Resolve triggers and schedules the functions registered with .and then
So we tin can employ promises to exercise asynchronous work, and to exist sure that we can handle any result from those promises. That is the value proposition. If y'all want to know more than about promises you lot can read more than about them here and here.
When nosotros employ promises, nosotros chain our functions onto the promise to handle the different scenarios.
This works, but we still need to handle our logic within callbacks (nested functions) once we get our results back. What if we could use promises but write synchronous looking code? It turns out we tin.
Async/Await
Async/Expect is a way of writing promises that allows us to write asynchronous lawmaking in a synchronous fashion. Let's have a look.
const getData = async () => { const response = await fetch("https://jsonplaceholder.typicode.com/todos/1") const data = look response.json() panel.log(data) } getData()
Nothing has changed nether the hood here. We are still using promises to fetch data, only now information technology looks synchronous, and we no longer have .so and .catch blocks.
Async / Await is actually just syntactic sugar providing a way to create code that is easier to reason about, without irresolute the underlying dynamic.
Let'southward have a expect at how it works.
Async/Await lets us use generators to pause the execution of a function. When we are using async / wait nosotros are not blocking because the function is yielding the command back over to the main program.
Then when the promise resolves we are using the generator to yield control dorsum to the asynchronous function with the value from the resolved promise.
You tin can read more here for a smashing overview of generators and asynchronous code.
In issue, nosotros tin can now write asynchronous lawmaking that looks like synchronous code. Which means that it is easier to reason nearly, and nosotros can use synchronous tools for fault treatment such as try / grab:
const getData = async () => { endeavour { const response = look fetch("https://jsonplaceholder.typicode.com/todos/1") const data = await response.json() console.log(information) } catch (err) { console.log(err) } } getData()
Alright. So how exercise nosotros use it? In society to use async / look nosotros need to prepend the function with async. This does not make information technology an asynchronous function, information technology merely allows usa to employ await inside of information technology.
Failing to provide the async keyword volition result in a syntax error when trying to utilize await within a regular function.
const getData = async () => { console.log("We tin can use expect in this role") }
Because of this, nosotros can not employ async / await on elevation level code. But async and wait are however just syntactic sugar over promises. So nosotros can handle top level cases with promise chaining:
async part getData() { permit response = expect fetch('http://apiurl.com'); } // getData is a hope getData().then(res => panel.log(res)).catch(err => console.log(err);
This exposes another interesting fact virtually async / await. When defining a function every bit async, it will e'er render a promise.
Using async / wait can seem like magic at get-go. But similar whatsoever magic, it'due south simply sufficiently advanced technology that has evolved over the years. Hopefully now you have a solid grasp of the fundamentals, and can use async / look with confidence.
Decision
If you fabricated it here, congrats. You just added a key piece of noesis about JavaScript and how it works with its environments to your toolbox.
This is definitely a confusing subject, and the lines are not always clear. Just now yous hopefully have a grasp on how JavaScript works with asynchronous code in the browser, and a stronger grasp over both promises and async / await.
If you enjoyed this article, yous might besides savor my youtube aqueduct. I currently accept a web fundamentals series going where I go through HTTP, building web servers from scratch and more.
There'due south also a series going on edifice an unabridged app with React, if that is your jam. And I program to add much more content here in the future going in depth on JavaScript topics.
And if you want to say hi or conversation nigh web evolution, yous could always reach out to me on twitter at @foseberg. Thanks for reading!
Acquire to code for free. freeCodeCamp's open up source curriculum has helped more than 40,000 people go jobs as developers. Get started
mcdowellseestrocces.blogspot.com
Source: https://www.freecodecamp.org/news/async-await-javascript-tutorial/
0 Response to "Going Through Javascript Basics Again After Finishing It Freecodecamp"
Postar um comentário